git basics (quick reference)
We go over a small guidance into git / github / commits / adding files and deleting them.
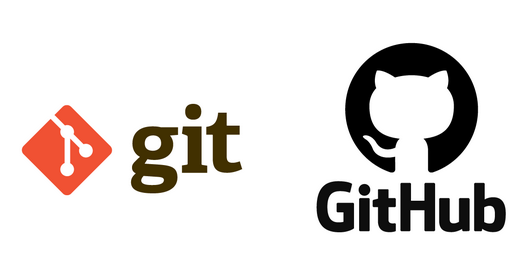
Quick review of how to use git.
- Make your repository: https://github.com/new
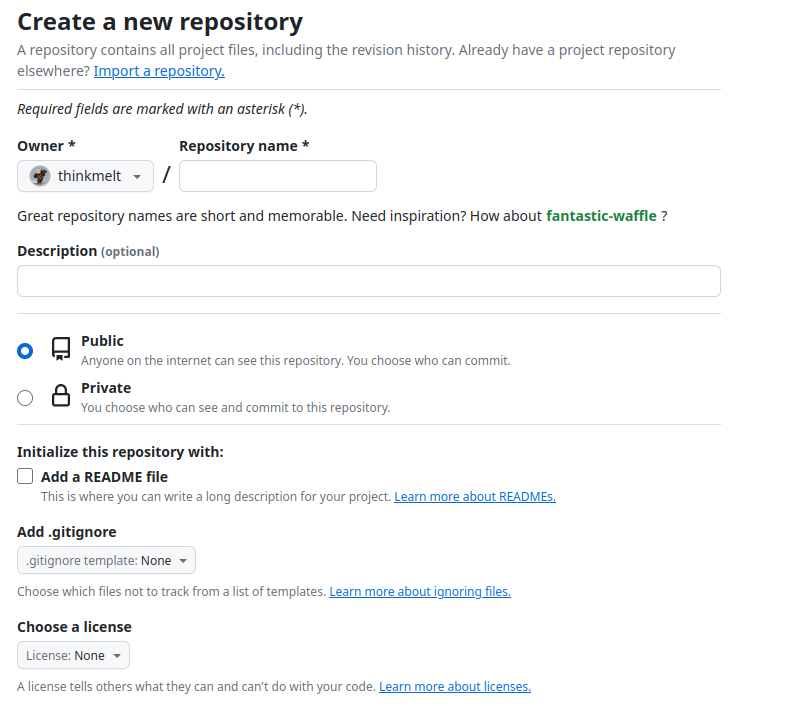
You will be presented with a command line option - but it is pretty basic:
echo "# test" >> README.md
git init
git add README.md
git commit -m "first commit"
git branch -M main
git remote add origin https://github.com/thinkmelt/test.git
git push -u origin main
Working through this command set as not a lot is explained as to what is going on here:
git init
Will produce the .git hidden directory
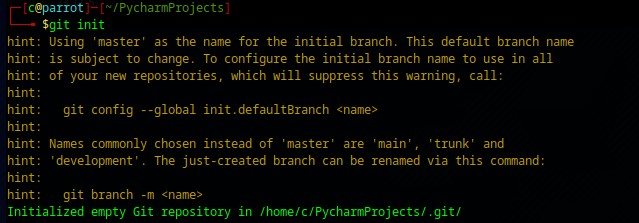
Adding files:
git add README.md # Add a single file
git add -A # Recursively add everythigng
git add --all # Recursively add everything.
Once you have added to your git list, you need to 'commit' it as in:
git commit -m "first commit"
Understanding that 'git commit' is a 'index snapshot' of the files at that time. But only locally to your .git directory tracker.
And then sync it upstream with:
git push -u origin main
# Please not
Gotcha: github now has added token authorization that you need to complete before pushing to anything and it requires its own installation. 'Console' logins are now deprecated. So:
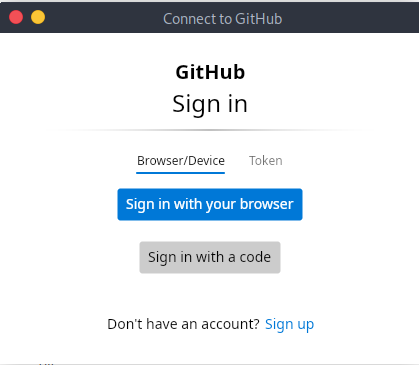
Which if you are holding cookies inside your browser, and are currently logged into github you will now get:
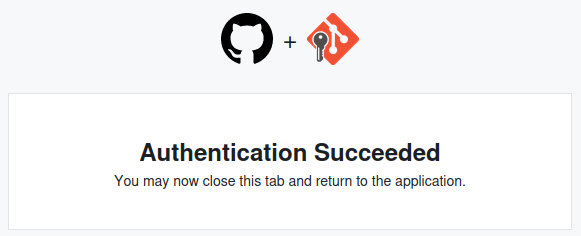
Caution: Removing files vs .gitignore
Typically one can make a .gitignore put in your list of wildcards, and it will not sync to your online repository (but remain local). However one must use a lot of caution as it would presume that 'git rm' indicates that you wanted it removed from the online repository - it just deletes the file if you had issued a 'rm' command!
- Let's presume that we want to remove 1 file from our git and then push it back again.
git rm places.txt && git commit -m "Next Branch"
We will see the following:
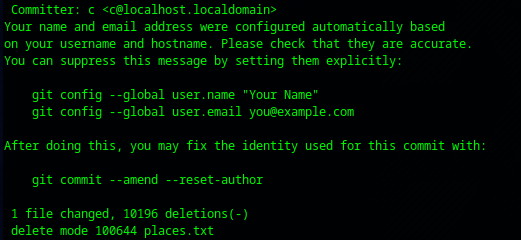
git push -u origin
However it has purged it from both the local repository AND the online repository - Ouch! The devil is in the details. 'git rm' removes it from the git local repository listing. 'git commit' actually does the delete!
Understanding git synchronizing against a fresh .gitignore
Now that we know to use a LOT of caution pairing the 'git rm' with the 'git commit' we will
git add .
git commit -m "Readded files"
git push -u origin
git rm -r --cached .
# add all files as per new .gitignore
git add .
# now, commit for new .gitignore to apply
git commit -m ".gitignore is now working"
git push -u origin
Listing Files: git ls-files
git uses a complex indexing system which you can read more about here:
In essence we can:
git ls-files #or
git ls-files --stage
For a more detailed listing:
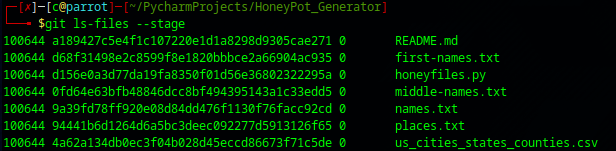
Listing and handling Commits:
One may list all the historical commits with:
git log # It is very detailed therefore
git log | grep commit
One may switch to a commit with:
git checkout -f cbc4... long string
You will get a long update as in:
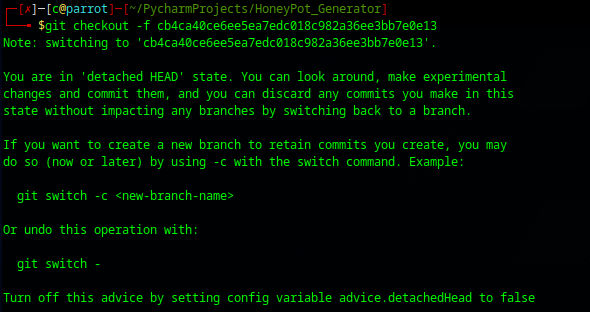
Making a branch:
git push --set-upstream origin newbranch
This will make a branch / fork off the origin for new branch.
When you examine the main page it will now show the branch:
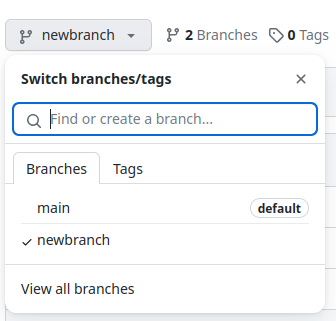