Python 3.13 Dockerfile building and testing.
In this roll-your-own article we go over setting up our own python container that has rich support for specialized python applications.
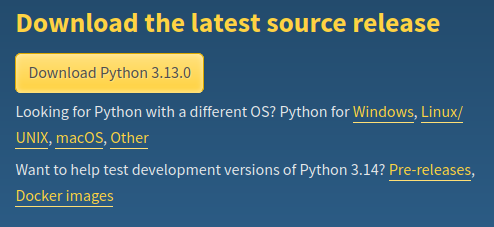
Python can get messy because if you have advanced packages - your python software may not run - so put it into a Docker container and virtualize it!
We make our 'Dockerfile' in a fresh directory and put into it:
FROM ubuntu:latest
RUN apt-get update
RUN apt-get install -y software-properties-common --fix-missing
RUN apt-get install wget htop pkg-config nano build-essential zlib1g-dev pip openssl -y
RUN apt-get install libssl-dev libncurses5-dev libsqlite3-dev libreadline-dev libtk8.6 libgdm-dev libpcap-dev -y
RUN wget https://www.python.org/ftp/python/3.13.0/Python-3.13.0.tar.xz
RUN tar -xvf Python-3.13.0.tar.xz
WORKDIR Python-3.13.0
RUN chmod +x ./configure && ./configure && make -j$(nproc) && make install -j$(nproc)
WORKDIR /
RUN python3 -m pip install --upgrade pip
RUN python3 -m pip install --trusted-host pypi.org mysql-connector-python
RUN python3 -m pip install --trusted-host pypi.org ephem certifi colorama reportlab
ADD /python /python
WORKDIR python
CMD python3 stock_tabler.py
One can stand this up for a image with:
docker build . -t python_base
One can see it does build a fairly large image - but it is comprehensive:

- Reviewing what is happening here - effectively we are building from a Ubuntu image and then calling apt-get to install a pile of software that natively does not come with it.
- We download and build our own Python-3.13.0 from scratch, then we install via python3 -m pip install all the dependencies that are required.
- After that we copy our /python to /python which holds our software.
- Finally it has a generic command which will be called each time it is run.
CMD python3 stock_tabler.py
If we need to inspect the container we can fire up a interactive instance as in:
docker run -it python_base:latest /bin/bash
And now we can actually schedule cron to run this at a interval:
crontab -e
0 0 * * * docker run python_base:latest
If we want to save this image to move to another machine we can simply:
docker image save -o python_base.tar python_base:latest
Solving a credential failure:
Sometimes you cannot pull your image because your docker login is corrupted this will fix it.
gpg --generate-key
pass init <generated gpg-id public key>
From here you can do another docker test pull.
docker pull hello-world
When you being building it will take some time - on a 12-core push it still took about 3 minutes to build everything.
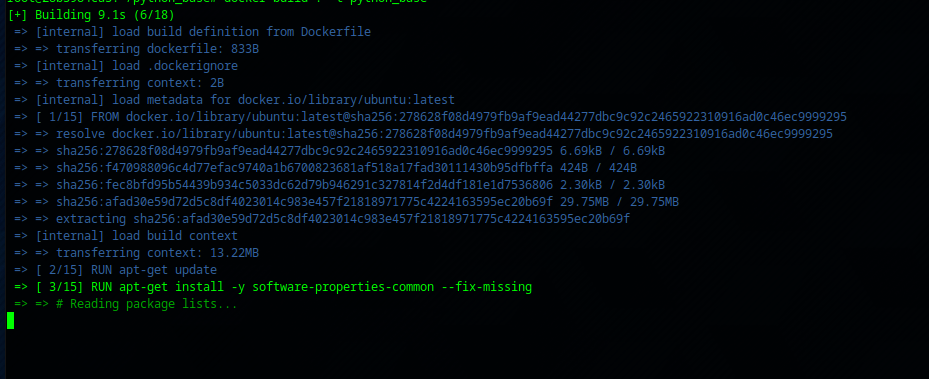
Getting data out from a temporary running container.
- There can be an issue of a temporarily running container which generates files but does not stay running.
- This is accomplished by using a named volume.
- A named volume is accessible by any participating docker container AND the root system.
docker volume create --driver local -o o=bind -o type=none -o device="/root/nms" nmsvol
Now when a container runs it can mount and access, read from and write to this volume:
docker run -d -v volume-data:/data --name nginx-test nginx:latest
Building an image with no-cache.
- Because there are a LOT of misguidance on how to do this, this is how to build without caching to force a rebuild if images are corrupted etc:
docker build --no-cache -t <image_name> .
You can also clean out the build-cache which is quite hidden but visible with:
docker system df
You will see the build cache

In this instance we can see we have 12 images but actually the build-cache holds 44 (12 active) taking up an additional 846.3 MB. To clean this we can use:
docker builder prune
If that is not strong enough go up one level:
docker system prune