Setting up an actual Working Eclipse IDE for Raspberry Pi-Pico Development with OpenOCD
We *actually really* get Eclipse to work with OpenOCD and a Raspberry Pi-Pico for rapid development.
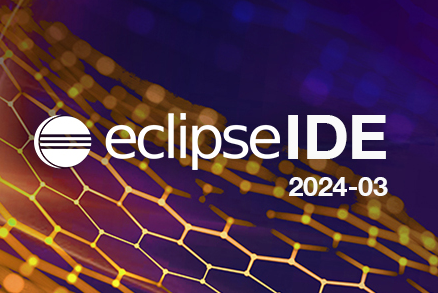
Before we start! Music of the day: Deep Programming Music
- End result. 1-3 second compile / program / reset / run cycles.
- Stepping-debugging was not completely successful but it is close.
- This guide is for a Linux build.
Step 0: Install your basics..
sudo apt update -y && sudo apt install build-essential -y
sudo apt install gcc cmake gcc-arm-none-eabi -y
# optional extras suite to taste..
sudo apt install manpages-dev -y
sudo apt install g++ make -y
Step 1: Buy (or convert-build) a Raspberry Pi-Pico Probe:
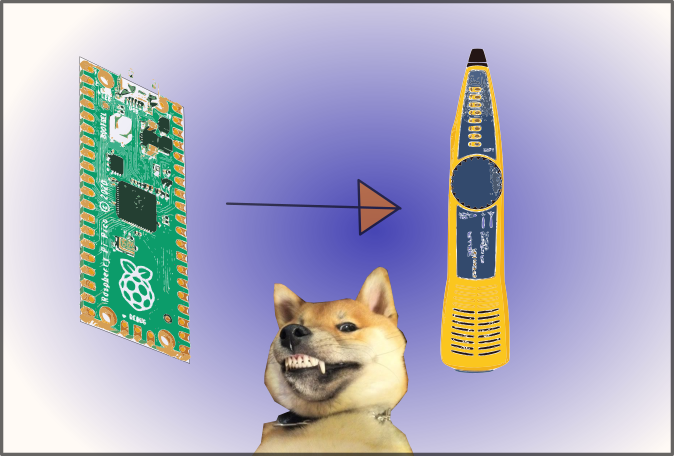
Step 2: Make sure it is running cleanly and programming a target:
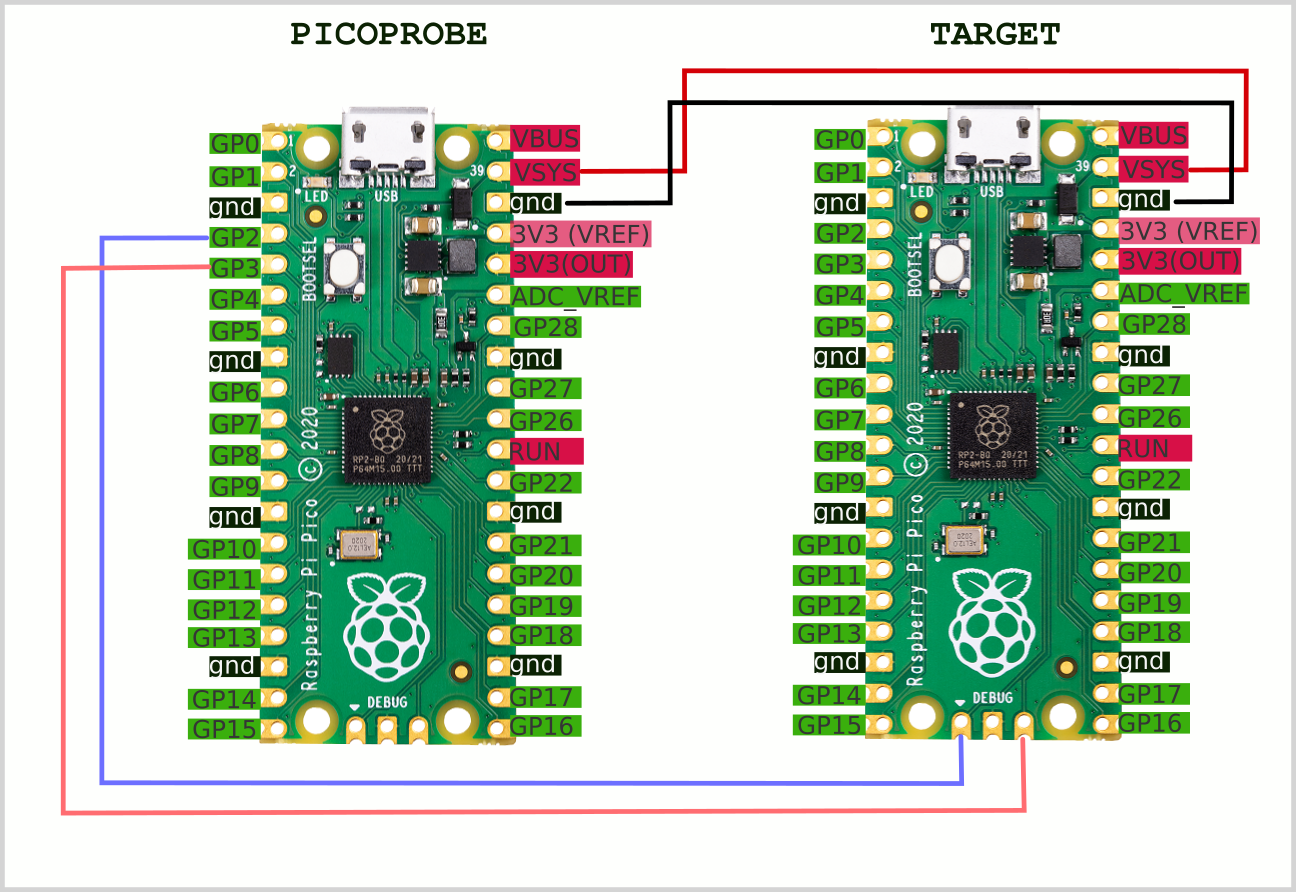
- At this point you should have the 'electronic exterior' running cleanly.
Step 3: Download and install Eclipse for Embedded Development. Â
- This guide is 'over-explained' as a standard, yet kept simple as possible for your best odds of success. Let's get started:
- It will download a .tar.gz file as in:
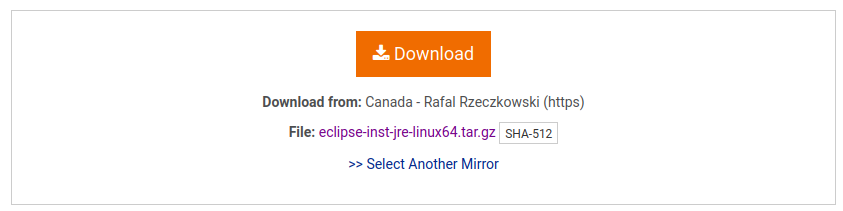
We can unpack it with:
tar -xvf eclipse-inst-jre-linux64.tar.gz
Which will make your 'eclipse-installer' directory:

Then run the installer:
cd eclipse-installer && ./eclipse-inst
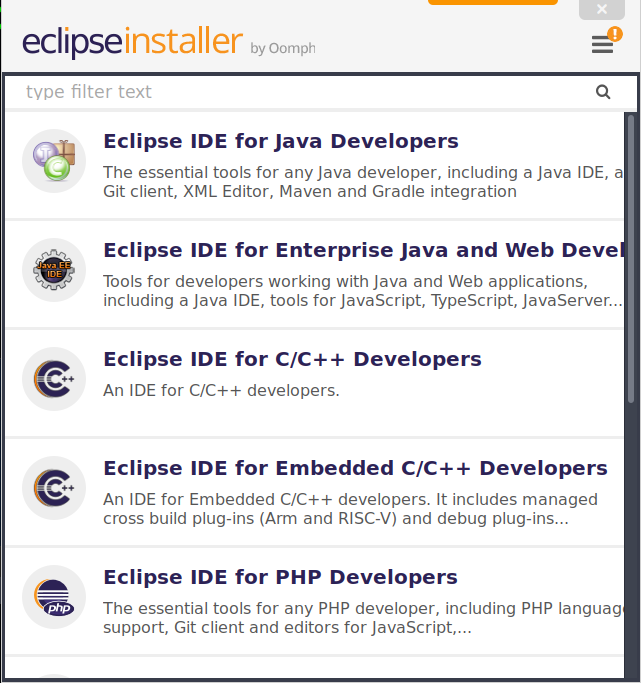
- We will select the Eclipse IDE Â for Embedded C/C++ Developers
- Set your install directory...
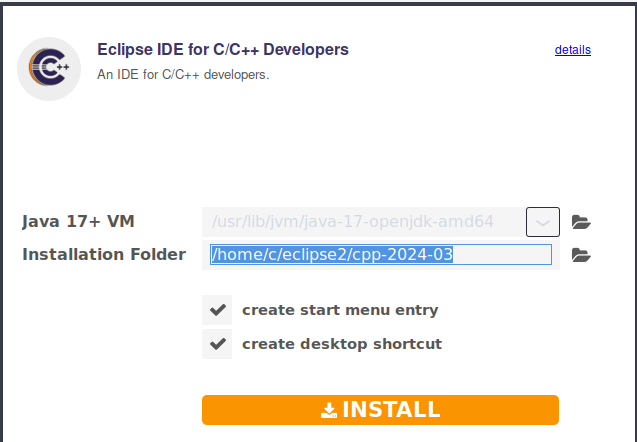
And it will install:

And launch.. the launcher is sorta buried for me it came out as a directory structure of:
/home/c/eclipse2/cpp-2024-03/eclipse
On boot it will banner:
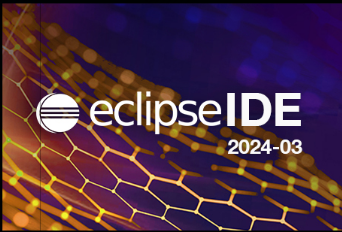
- Set a directory as a workspace, a workspace is for all your projects:
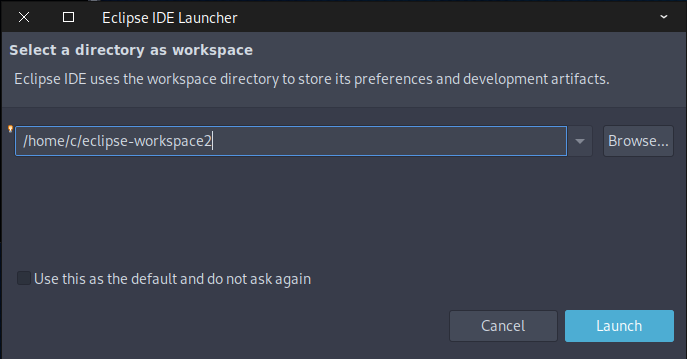
- You will get your inital welcome screen:
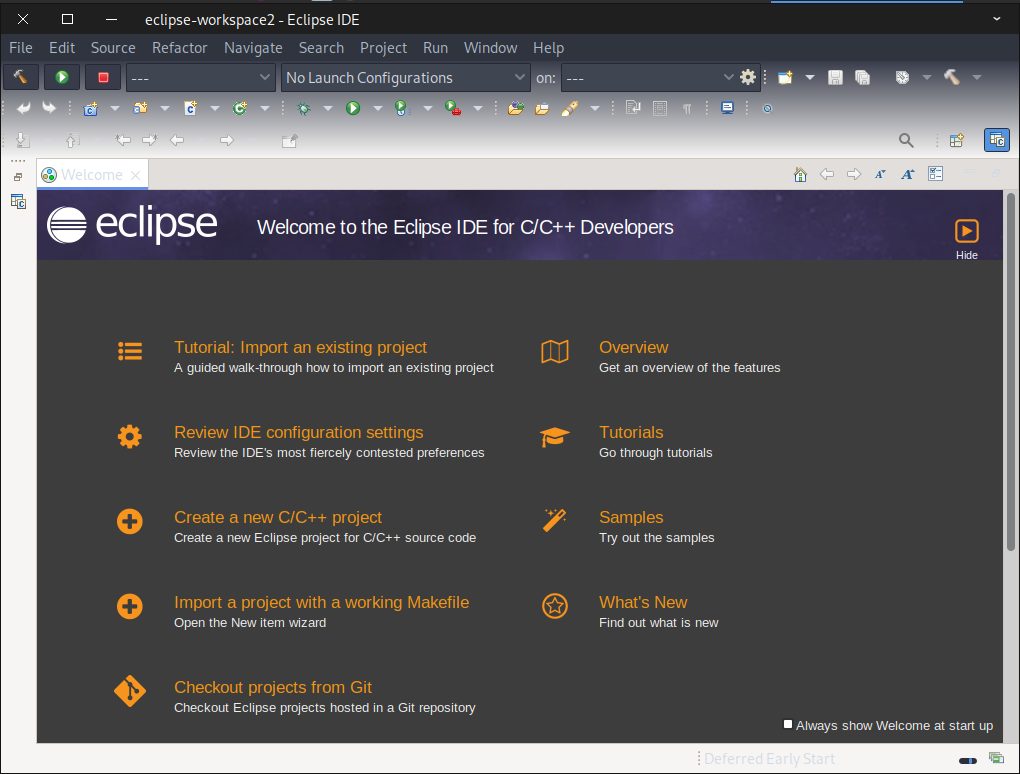
Step 4: Adding in the add-ins you will need.
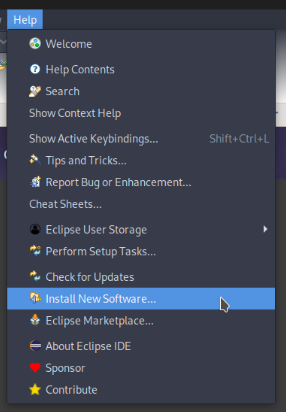
- Add cmake support:
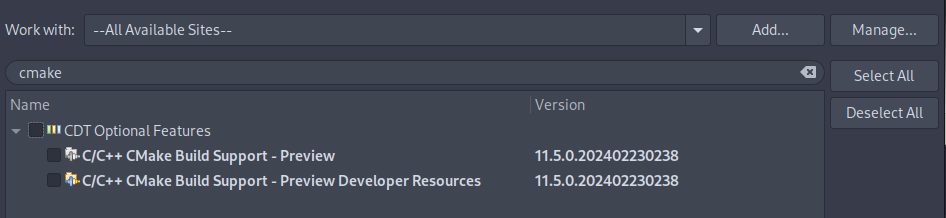
- In the corner it will show the software install / update and then do a restart...

- Next we installed everything embedded as in (the key one is CMSIS Packs)
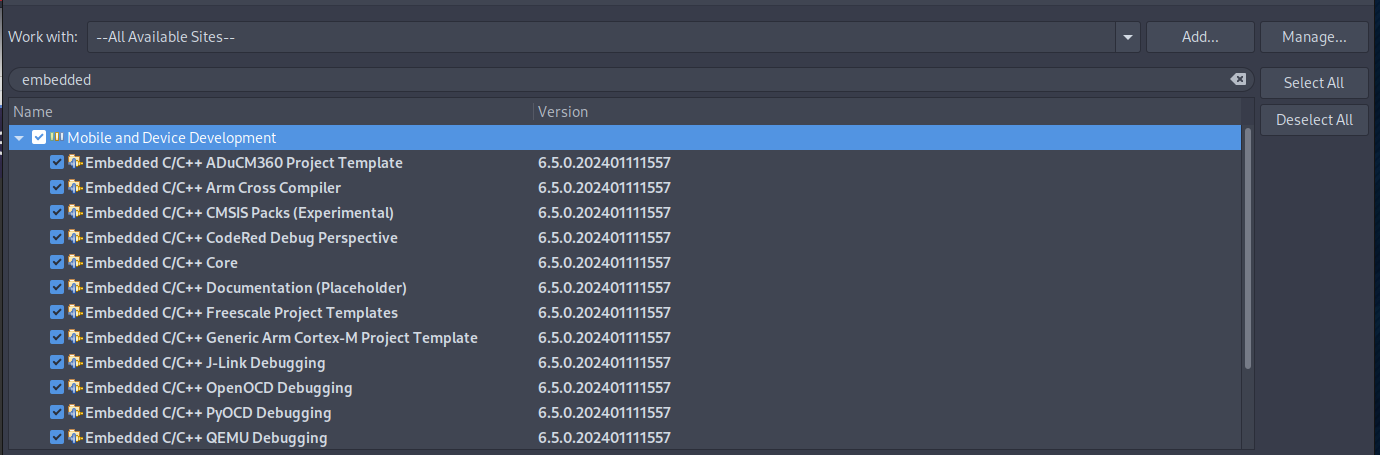
Step 5: Getting it to import a cmake pico-type project.
- Make sure you have the pico-sdk downloaded somewhere.
- Where did you install it - note that, for me it was installed at:
/home/c/coding/pico-sdk
- Now we will make a new pico type project in a new folder make the following CMakeLists.txt file:
cmake_minimum_required(VERSION 3.13)
include(/home/c/coding/pico-sdk/pico_sdk_init.cmake) #<- Note here
project(test C CXX ASM)
pico_sdk_init()
add_executable(hello_world hello_world.c)
target_link_libraries(hello_world pico_stdlib)
pico_add_extra_outputs(hello_world)
pico_enable_stdio_usb(hello_world 1)
pico_enable_stdio_uart(hello_world 0)
- NOTE - the include above is set to where lives your pico-sdk namely it's root pico_sdk_init.cmake file. Â Adjust it to where you put yours..
- Now we will add the hello_world.c program as noted above on line:
add_executable(hello_world hello_world.c)
In our hello_world.c we will put some boilerplate code:
#include <stdio.h>
#include "pico/stdlib.h"
#include "hardware/gpio.h"
int main()
{
const uint apin = 0;
gpio_init(0);
gpio_init(5);
gpio_init(1);
gpio_init(2);
gpio_init(25);
gpio_set_dir(0, GPIO_OUT);
gpio_set_dir(1, GPIO_OUT);
gpio_set_dir(2, GPIO_OUT);
gpio_set_dir(25, GPIO_OUT);
stdio_init_all();
while (true)
{
gpio_put(0, 1);
gpio_put(1, 1);
gpio_put(2, 1);
gpio_put(25, 1);
printf("Hello, world!\n");
sleep_ms(300);
gpio_put(0, 0);
gpio_put(1, 0);
gpio_put(2, 0);
gpio_put(25, 0);
sleep_ms(300);
}
return 0;
}
- Now you have only two files in this directory as in:
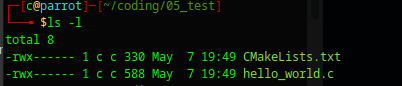
- Ready? Now lets make this pico-sdk / eclipse compatible. Inside the directory issue this command:
cmake -G "Eclipse CDT4 - Unix Makefiles" ./
If it runs cleanly you will have a pile of files and the following output:
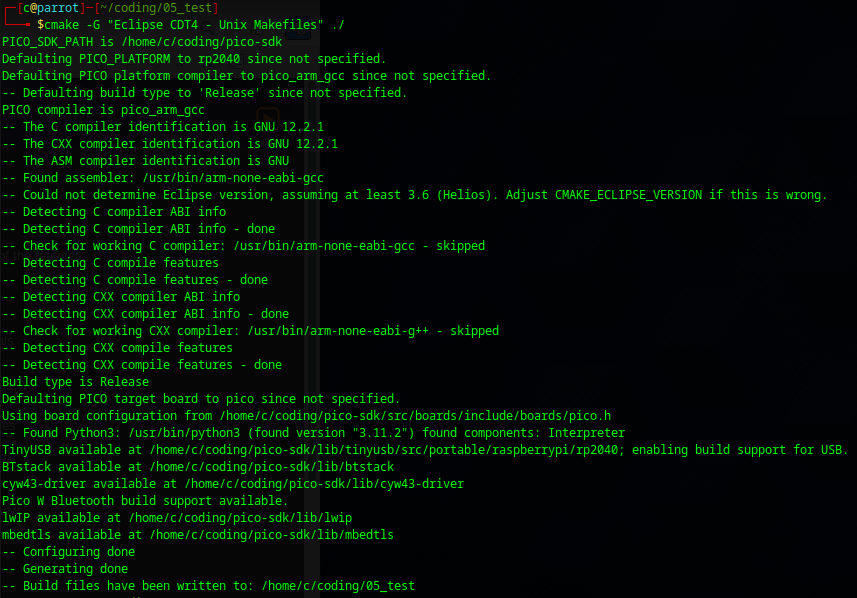
Next from inside eclipse we will 'Open Projects from File System'
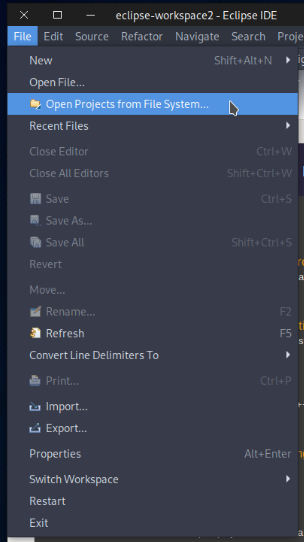
We set the directory where the above run:
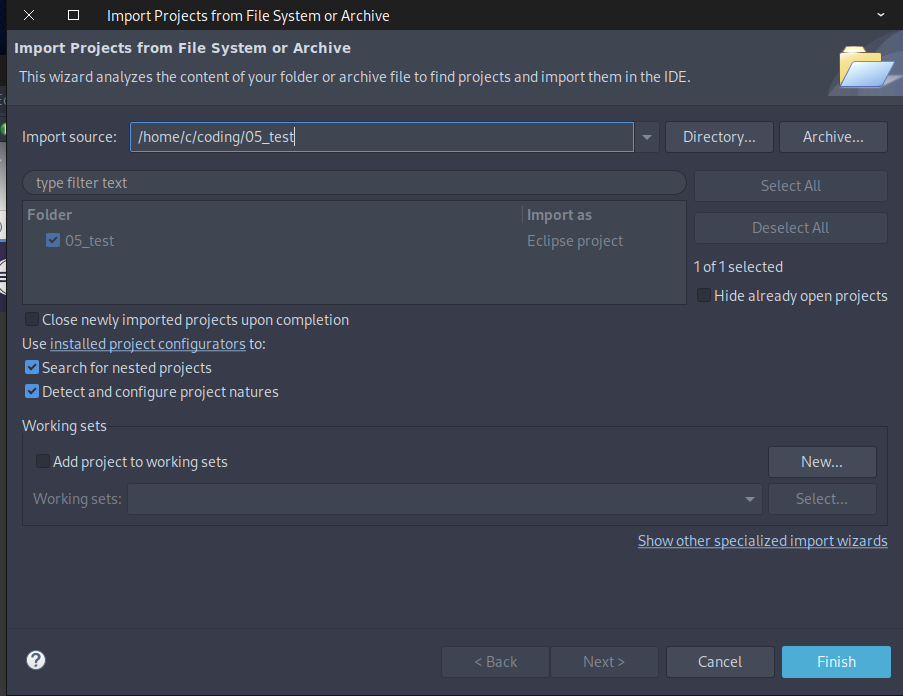
- The Indexer does a lot - in fact it pulls an entire copy of the pico-sdk into the current project directory...

- How do you know it worked correctly? If you hold your mouse over a command it will show the suggestor for it.
- Also you will have a structure of proper directories on your left.
- Colors look a lot better with 'Dark Theme'
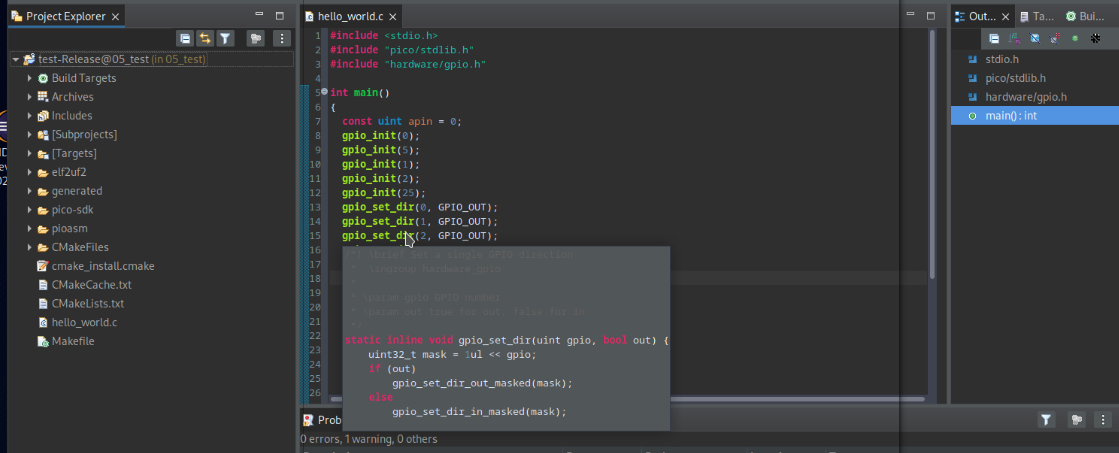
Step 6: Clean and Fast Transfer Compiling.
- Right click inside your hello_world.c program and we will  make a run-configuration that will work with the CMSIS configured Pico-Probe (its not there by default)
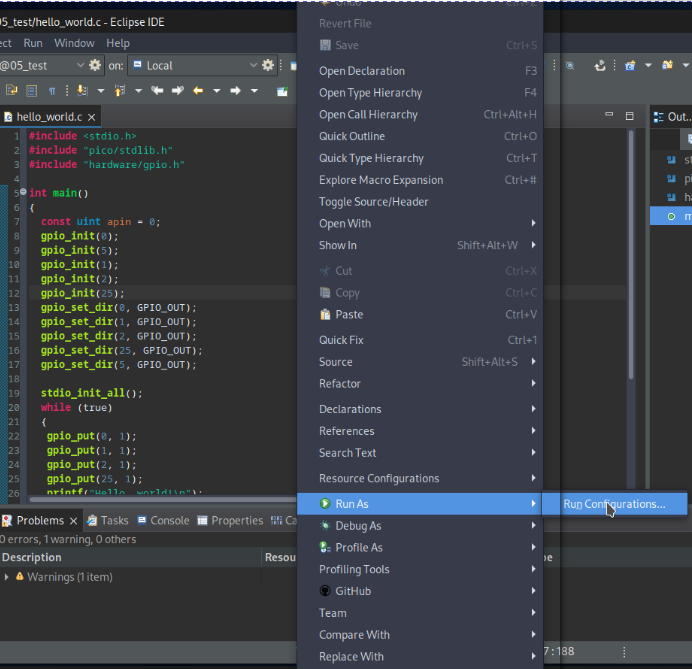
- Double click on the GDB OpenOCD Option (to make a new run option)
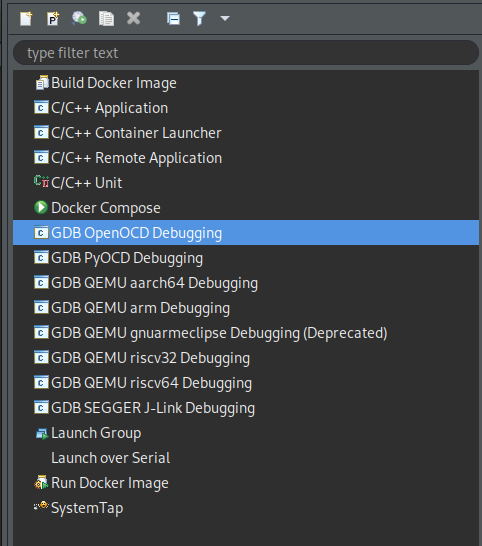
For the main tab we set the C/C++ application as:
/usr/bin/arm-none-eabi-gcc
- Which should of been installed in Step 0:
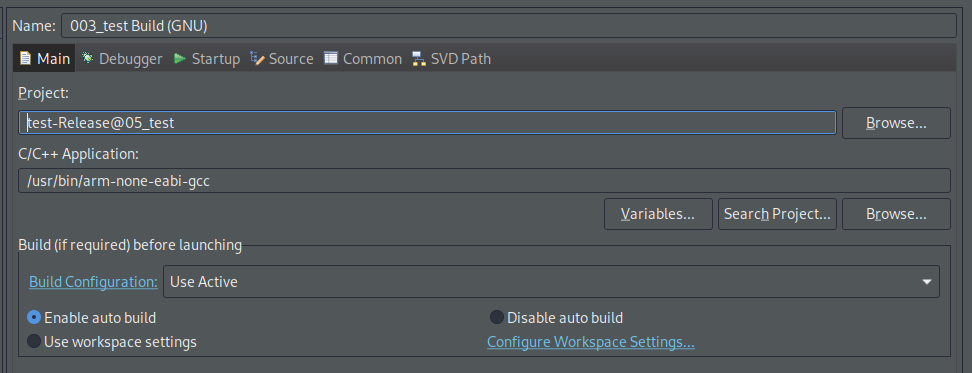
For the debugger tag we set it up as:
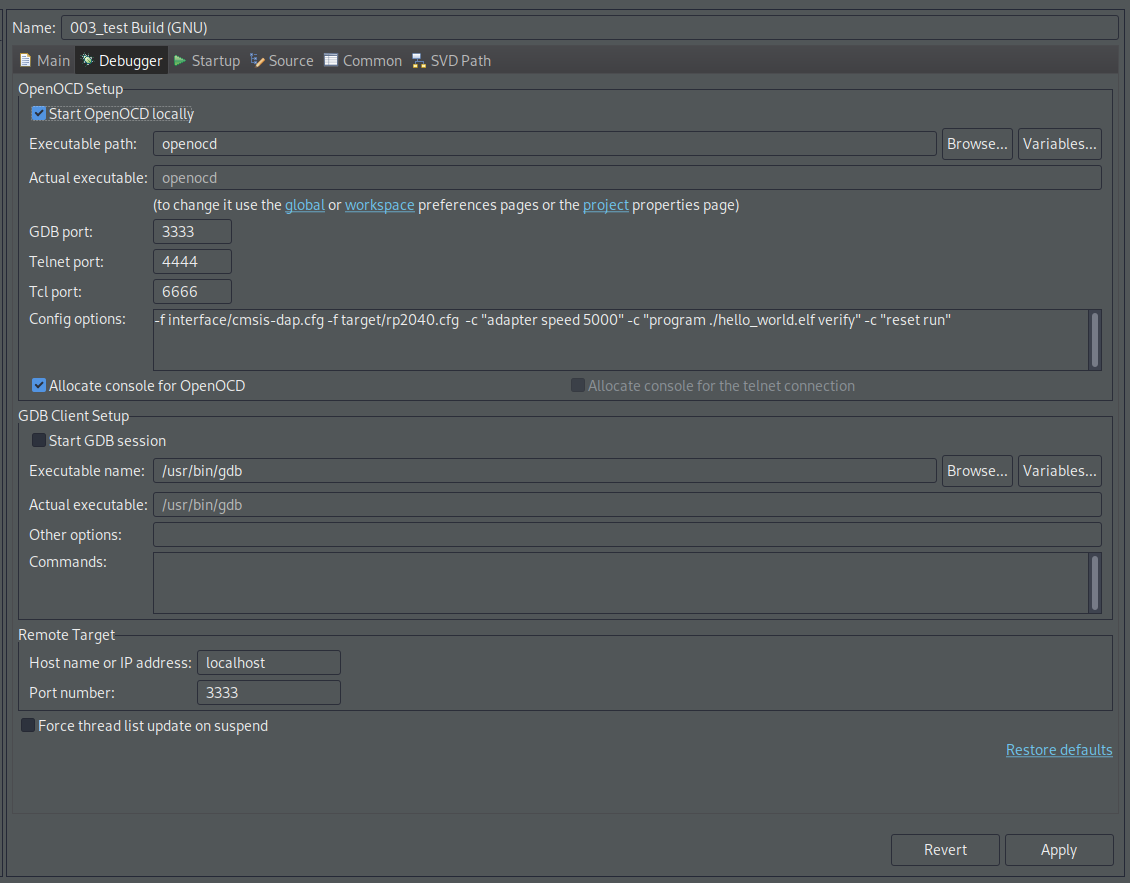
- Note the OpenOCD option is setup as:
-f interface/cmsis-dap.cfg -f target/rp2040.cfg -c "adapter speed 5000" -c "program ./hello_world.elf verify" -c "reset run"
-f interface/cmsis-dap.cfg (will work if you followed step 1 accurately)
-f target/rp2040.cfg (to program another rpi2040 raspberry pico board)
-c "program ./hello_world.elf verify"
- Program the hello_world.elf to the rpi2040 and verify it's good.
-c "reset run"
- Reset the chip and run the program.
Even though we require gdb to be installed we leave it clicked off as:
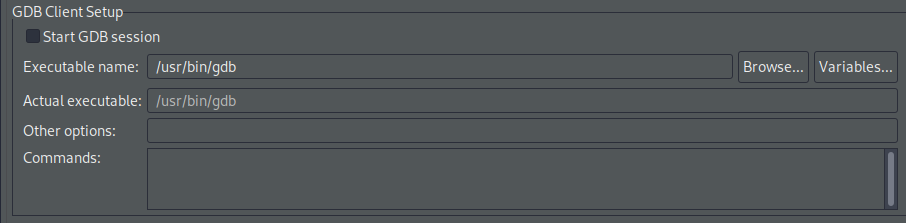
Sweet sweet victory when this stuff works!
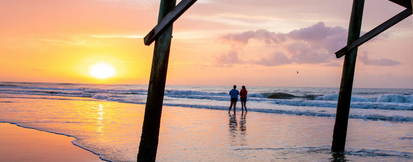
You should see an output as:
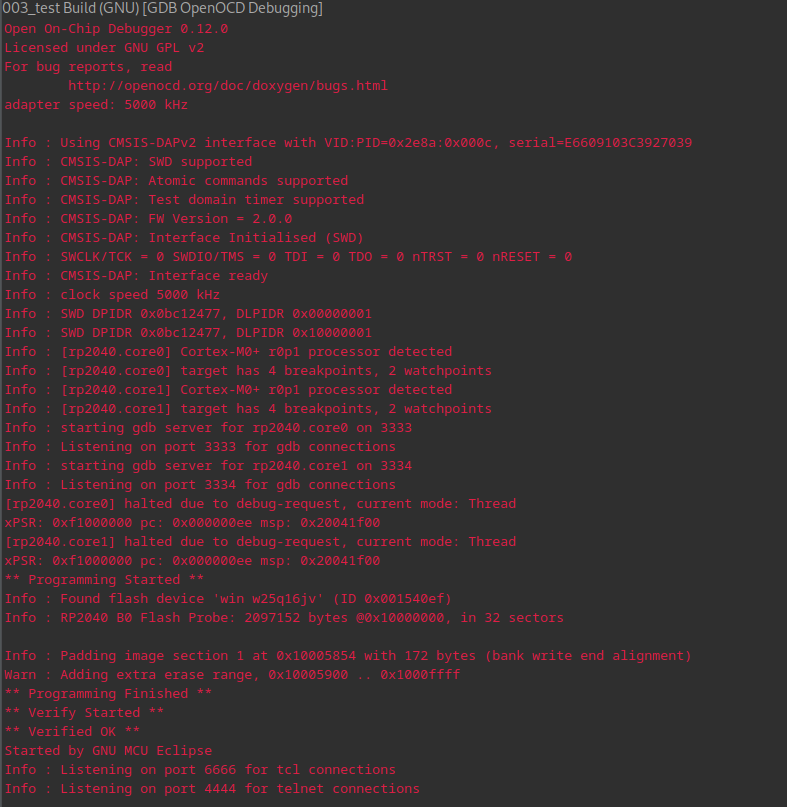
Summary:
- It has been my experience that getting accurate and usuable information of getting anything to work in Eclipse, much less rapid programing a Raspberry Pi Pico from a strictly Linux environment is pretty much non-existant.
- Sites can be found that cover one or the other but not the whole process, and not at this explicit detail. Â It is typical that advanced developers assume you know the 20 tiny critical sub-steps, but a often and realistically we do not!
- It is very close - this almost has step-debugging working, but this in of itself is really good and hopefully will help lots of struggling coders trying to piece together a very complex software development ecosystem.
Stepper-Debugging HAS been successfully accomplished with CLion please see:
- It downloads, programs, resets and runs the fresh program in about a second if your speed is up, that is really good!
- Giving big thanks!